Want to improve how your app shows documents? A good document viewer can make reading and working with files easier for users. This guide will show you how to make a document viewer and slider using Filestack, a file-handling tool.
Document viewers are critical in apps today. They let users see different types of files, such as PDFs and spreadsheets, without needing extra software. A good document viewer can make a big difference if you’re making a system for managing files or a tool for sharing documents.
In this guide, we’ll explain what a document viewer is and why it’s useful. We’ll show you how to make your own using Filestack. We’ll start by getting an API key, then set up your environment, and finally build a sample app. Let’s get started!
What is a document viewer?
A document viewer helps you open and view digital documents on your computer or online. It lets you see files like PDFs, Word documents, and presentations without needing the specific programs that created them.
Here are some everyday examples of document viewers:
- Adobe Acrobat Reader
This is a popular tool for opening and reading PDF files. It’s useful for reading documents, forms, and manuals.
- Microsoft Word Viewer
This free tool lets you open and view Word documents even if you don’t have Microsoft Word on your computer. It helps read Word files without the full program.
- Google Docs Viewer
This tool lets you view different types of documents, like PDFs and Word files, right in your web browser. It’s useful for quickly looking at Gmail or Google Drive attachments without downloading them.
- Microsoft PowerPoint Viewer
Similar to Word Viewer, this tool lets you view PowerPoint presentations without needing the full PowerPoint program. It helps view presentations on computers that don’t have PowerPoint installed.
Document viewers are important for everyday tasks like reading reports, checking contracts, and sharing information. They make it easy to view documents without needing specific software, which is great for personal and work use.
What are the benefits of a document viewer?
👉A document viewer makes it easy to look at files without installing special software. It helps you view PDFs, Word docs, and spreadsheets from anywhere. You can also share files quickly through email or links.
👉One of the main benefits is its convenience. You can access and view files from anywhere at any time, which is great for working remotely or collaborating with others far away.
👉Another advantage is how it simplifies things. You don’t need different apps for different file types. Everything is in one place, making it faster to find what you need.
👉It also helps with security. You can protect files with passwords and control who can see them. This keeps your information safe and only accessible to the right people.
Applications
Document viewers are used in many ways in real life. In businesses, they help people see reports and presentations easily. This means employees can look at important information without special software.
✔️In schools, document viewers show textbooks, notes, and research papers. This makes it easier for students and teachers to share and learn together.
✔️In law, document viewers are used for legal papers and case files. They help lawyers manage lots of information.
✔️In healthcare, document viewers show medical records and images. This helps doctors see and plan treatments.
How do you develop a document viewer with Filestack?
Here are the steps to develop a document viewer with Filestack.
Getting API key
- Create an account at Filestack.
- Verify your email and log in to the account.
- Navigate to the dashboard and get the API Key.
Setting up the environment
Open the command prompt and create a directory by using the following command:
mkdir filestack-document-viewer
Open the directory in the Visual Studio Code by using the commands given below:
cd filestack-document-viewer
code .
When the folder opens in the Visual Studio Code, create index.html file.
Now, let’s move to building our sample application.
Building the sample application
HTML Structure
<!DOCTYPE html>
<html>
<head>
<title>Filestack Document Preview App</title>
<script src="https://static.filestackapi.com/filestack-js/3.x.x/filestack.min.js"></script>
<style>
</style>
</head>
<body>
<div class="container">
<h1>Filestack Document Preview App</h1>
<button id="uploadBtn">Upload Document</button>
<div id="documentPreview"></div>
</div>
</body>
</html>
- The code starts with the standard HTML5 doctype declaration (<!DOCTYPE html>).
- The <html> tag contains the entire document.
- The <head> section includes the title of the app and a link to the Filestack JavaScript library.
- The <style> section defines the app’s styling, including fonts, colors, layout, and button styles.
- The <body> tag contains the visible content of the app.
- Inside the body, there is a <div> with the class “container” that wraps the main content of the app.
- Inside the container, there is an <h1> heading with the text “Filestack Document Preview App”.
- A <button> element with the id “uploadBtn” is used to trigger the document upload process.
- Another <div> with the id “documentPreview” is used to display the document preview.
CSS Styling
body {
font-family: Arial, sans-serif;
background-color: #EF4A25;
margin: 0;
padding: 0;
text-align: center;
}
.container {
max-width: 600px;
margin: 50px auto;
padding: 20px;
background-color: #fff;
border-radius: 10px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
}
h1 {
color: #333;
font-size: 24px;
margin-bottom: 20px;
}
#uploadBtn {
background-color: #EF4A25;
color: #fff;
border: none;
padding: 10px 20px;
font-size: 16px;
border-radius: 5px;
cursor: pointer;
}
#uploadBtn:hover {
background-color: #0056b3;
}
#documentPreview {
margin-top: 20px;
}
- The body style sets the font family, background color, margin, padding, and text alignment for the entire document.
- The .container style sets the maximum width, margin, padding, background color, border radius, and box shadow for the main container of the app.
- The h1 style sets the color, font size, and margin bottom for the main heading of the app.
- The #uploadBtn style sets the background color, text color, border, padding, font size, border radius, and cursor for the upload button. It also defines a hover effect when the button is hovered over.
- The #documentPreview style sets the margin top for the document preview container, which contains the uploaded document preview.
JavaScript Functionality
<script>
const client = filestack.init('ADD YOUR API KEY HERE');
let currentMode = 'preview';
document.getElementById('uploadBtn').addEventListener('click', () => {
client.picker({
accept: [
'application/pdf',
'application/vnd.ms-powerpoint',
'application/vnd.openxmlformats-officedocument.presentationml.presentation',
'application/vnd.ms-excel',
'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet',
'application/msword',
'application/vnd.openxmlformats-officedocument.wordprocessingml.document',
'application/vnd.oasis.opendocument.text',
'application/vnd.oasis.opendocument.presentation',
'image/gif',
'image/tiff',
'image/jpeg',
'image/png',
'text/html',
'text/plain',
'application/illustrator',
'image/vnd.adobe.photoshop'
],
onUploadDone: (res) => {
const handle = res.filesUploaded[0].handle;
generateDocumentPreview(handle);
}
}).open();
});
function generateDocumentPreview(handle) {
const documentContainer = document.getElementById('documentPreview');
const previewUrl = `https://cdn.filestackcontent.com/preview/${handle}`;
const slideUrl = `https://cdn.filestackcontent.com/slide/${handle}`;
const toggleButton = document.createElement('button');
toggleButton.textContent = 'Toggle View';
toggleButton.addEventListener('click', () => {
if (currentMode === 'preview') {
documentContainer.innerHTML = `<iframe src="${slideUrl}" style="width: 100%; height: 500px;" frameborder="0"></iframe>`;
currentMode = 'slide';
} else {
documentContainer.innerHTML = `<iframe src="${previewUrl}" style="width: 100%; height: 500px;" frameborder="0"></iframe>`;
currentMode = 'preview';
}
});
documentContainer.innerHTML = `<iframe src="${previewUrl}" style="width: 100%; height: 500px;" frameborder="0"></iframe>`;
documentContainer.appendChild(toggleButton);
}
</script>
- The <script> tag contains the JavaScript code for the app.
- The filestack.init() method initializes the Filestack client with an API key.
- The document.getElementById(‘uploadBtn’).addEventListener() method adds a click event listener to the upload button. When clicked, it opens a Filestack file picker.
- The generateDocumentPreview() function is called when a file is uploaded. It receives the file handle and generates a preview URL and a slide URL using Filestack’s CDN.
- Inside generateDocumentPreview(), a toggle button is created to switch between preview and slide view modes.
- Depending on the current view mode, the function dynamically updates the document container’s content with an <iframe> element displaying the document preview or slide.
Output
When you run the code, it will show the below screen:
Click on the Upload Document button and choose the desired PDF like below:
When you click on the Upload button, you will be able to view the document under:
Click on the Toggle View button to enable the sliding option.
To view the final code, please go to this GitHub link: https://github.com/devayesha23/FilestackDocumentViewer
Check out the video of our application here:
Conclusion
Building a document viewer with Filestack can greatly improve how users interact with files in your app. It allows them to easily view various types of documents like PDFs and spreadsheets without needing extra software.
Filestack’s API makes it simple to integrate this feature into your app. Following this guide’s steps, you can create a document viewer that meets your users’ needs.
A document viewer is essential for apps that deal with files. It helps users view and manage documents, making it easier for them to access important information.
Filestack’s document viewer is cost-effective and secure, making it a great choice for developers. It allows you to enhance your app’s document management features without a lot of hassle.
Using Filestack to create a document viewer can improve the usability and functionality of your app, providing users with a better experience.
FAQs
Why should I choose Filestack document viewer?
Choose Filestack document viewer for easy integration, cost-effectiveness, and secure document management. Enhance user experience without complex software requirements.
Is it easy to integrate Filestack document viewer?
Integrating Filestack’s document viewer is easy. You can follow simple steps to add it to your app without needing advanced technical skills.
What is the cost of integration for a document viewer?
The cost of integrating a document viewer depends on the specific features and customization needed for your app. However, Filestack offers flexible pricing plans to fit different budgets.
Is Filestack safe for creating a document viewer?
Filestack is safe for creating a document viewer. It offers secure file handling and protects user data, ensuring a safe experience for both developers and users.
Elevate your applications with the Filestack document viewer application today!
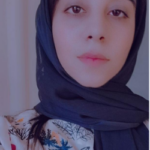
Ayesha Zahra is a Geo Informatics Engineer with hands-on experience in web development (both frontend & backend). Also, she is a technical writer, a passionate programmer, and a video editor. She is always looking for opportunities to excel in her skills & build a strong career.
Read More →