In today’s digital era, the use of mobile devices is continuously increasing thanks to their compact design, portability, and advanced functionality. However, this compact design and portability come at a price – limited built-in storage capacity. When developing mobile apps, such as iOS apps, developers often face several limitations due to these storage constraints. This is especially true for apps that require storing large amounts of data, such as multimedia like images and videos. That’s because images can take up a lot of space. Storing them on mobile devices’ limited built-in storage can lead to performance issues, negatively impacting the user experience. Thus, optimizing image management is essential for the seamless functionality and performance of the apps. This is where cloud storage and free images CDN come into play.
Cloud storage provides extra storage space to store images, while a free images CDN ensures quick and reliable image delivery in apps.
This article will discuss how the integration of cloud storage with an image CDN helps optimize iOS image management and enhance the user experience.
Harnessing Cloud Storage for iOS Images
Storing your iOS app images in cloud storage instead of directly on users’ devices can significantly enhance your app’s performance and overall user experience. With cloud storage, users don’t need to worry about images consuming lots of space on their devices. Moreover, developers can easily scale their image storage capacity as their app grows. Cloud storage also enables quick and seamless access to images from anywhere at any time.
You can also adopt a hybrid strategy for storing images. This involves leveraging both local and cloud storage to enhance the app’s overall performance and user experience. For instance, you can store or cache frequently accessed images locally on the user’s device while using a cloud backend as the primary repository for your multimedia files. This provides faster access to images and minimizes bandwidth usage without consuming lots of local storage space.
Major cloud storage providers
iCloud
iCloud is a reliable cloud storage service provided by Apple for its iOS, macOS, and other Apple devices. It offers seamless integration with Apple’s ecosystem and allows users to securely store and sync images and other data across Apple apps and devices.
Ease of integration
Since iCloud is the official cloud service provided by Apple itself, it seamlessly integrates with iOS apps. Developers can leverage Apple’s CloudKit to integrate iCloud services into their apps and interact with them. CloudKit provides APIs for storing and managing data, key-value storage, user authentication, and more.
Storage
iCloud provides up to 5 GB of free storage for all users. Beyond the free tier, Apple also offers premium subscription plans with storage ranging from 50 GB to 12 TB.
Dropbox
Dropbox is a widely known cloud storage service that is recognized for its ease of use and collaboration features. It enables users to store and organize different types of files, including various document types, images, and videos.
Ease of Integration
Dropbox provides Swift and Objective-C SDKs that allow for easy integration of Dropbox cloud storage functionalities into iOS apps. The SDKs have detailed documentation, which further eases the integration process. Developers can easily access features like file uploading, storage, download, synchronization, and sharing.
Storage
Dropbox offers a free tier with 2 GB storage. It also offers various paid subscription plans with more storage (starting from 2 TB).
Google Drive
Google Drive is another widely used cloud storage and file synchronization service provided by Google. The platform provides secure cloud storage for various file types, including documents, images, and videos.
Ease of Integration
Google Drive provides robust REST API for developers, enabling them to integrate Google Drive Storage into their apps with ease. Developers can leverage the API for file upload, storage, download, management, sharing, and synchronization.
Storage
Google Drive offers 15 GB of free storage for all users. Users can also purchase additional storage depending on their needs.
Choosing the Right Free Image CDN
A CDN, also referred to as a Content Delivery Network, is essentially a global network of servers and data centres distributed worldwide. It basically caches your app content, such as images, and stores their copies in its various servers located worldwide. Then, when a user accesses your app or web pages, the CDN delivers the images from the server nearest to the user. This significantly enhances the image delivery process by reducing latency. Moroever, CDN improves load times and enhances the user experience.
A free images CDN is like a regular CDN but specializes in optimizing, transforming, and delivering images. It is specially designed to serve images faster. In other words, using an image CDN service, you can deliver optimized images quickly to any user from any part of the world.
Factors to consider
Here are the key factors to consider when choosing a free images CDN for your iOS app:
Global distribution
If you’re creating an app for a global audience, choosing a free images CDN that has servers and data centres located worldwide is essential. The CDN will deliver the images from the server nearest to them, reducing latency and enhancing your app’s performance.
Feature set
In addition to global coverage, a good free CDN offers various robust features for quick image delivery. These include:
- Built-in tools and features for image optimization, such as compression, resizing, and image format conversion.
- Image transformation features, such as cropping, rotation, image filters and effects, watermarking, etc.
- Support for lazy loading techniques to prevent the loading of images until they are requested by the user.
- Robust built-in security features, such as access controls, token-based authentication, and secure connections (HTTPS).
Performance
When choosing a free images CDN, evaluate the following performance metrics:
- Page loading times: Assess the CDN’s ability to deliver fast page load times consistently.
- Latency: Evaluate how quickly the image CDN service delivers content from different geographical locations.
- Uptime: Assess the uptime of the CDN to ensure it’s reliable.
Ease of integration
Choosing an image CDN that integrates easily into your iOS app is crucial. It accelerates the development process and improves your app’s performance. For instance, using a CDN that offers a specialized iOS SDK optimized for iOS development allows for quick and seamless integration.
Popular image CDN Services
Some popular image CDN services include:
Filestack
Filestack is a complete file management solution offering powerful tools and APIs for online file upload, transformation, and delivery. It leverages a powerful CDN to deliver content, including images, to users worldwide. Filestack also offers a specialized iOS SDK that seamlessly integrates Filestack services into your iOS apps. When you upload a file with Filestack File Uploader, Filestack immediately returns a CDN URL that you can use to deliver files to your app users. Filestack also enables you to optimize and transform images through its Processing API using the CDN URL.
Cloudinary
Cloudinary is a popular cloud-based image and video processing platform. It enables users to upload and store images to the cloud and manage them efficiently. Cloudinary also has a powerful CDN that quickly delivers all your media assets (that you direct to Cloudinary) to your website or app users. It also allows you to quickly transform images to different formats and dimensions and apply filters and image enhancements.
ImageKit Image CDN
ImageKit is a popular image CDN service that offers fast CDN delivery. It also offers powerful real-time image optimization and transformation features, including compression, resizing, cropping, rotation, watermarks, and text overlays. ImageKit also offers an efficient media library with unlimited storage. Additionally, ImageKit has a specialized iOS SDK that is designed to simplify URL generation and file uploading in iOS apps.
Integrating your image CDN
Here are the key steps for integrating a free images CDN into your iOS app:
Uploading to cloud storage
If you’re looking to integrate cloud storage with an image CDN in your app, you first need to upload your iOS app images to your selected cloud storage. For instance, if you’re using Dropbox, you can use its Swift iOS SDK to upload images to the cloud. Once your image is successfully uploaded, you’ll get a permanent storage URL or identifier for the uploaded image. You’ll use this URL to access the image later.
Here is an example code for uploading images to Dropbox using its Swift iOS SDK and getting a shared link for the image:
import UIKit
import SwiftyDropbox
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Ensure that the image exists in the app bundle
guard let imageURL = Bundle.main.url(forResource: "example_image", withExtension: "jpg") else {
print("Error: Image file not found in app bundle.")
return
}
// Configure Dropbox access token
let accessToken = "YOUR_DROPBOX_ACCESS_TOKEN"
DropboxClientsManager.setupWithAppKey("YOUR_DROPBOX_APP_KEY")
// Upload image to Dropbox
let client = DropboxClientsManager.authorizedClient
let fileData = try? Data(contentsOf: imageURL)
if let client = client, let fileData = fileData {
let request = client.files.upload(path: "/example_image.jpg", input: fileData)
request.response { response, error in
if let error = error {
print("Error uploading image to Dropbox: \(error)")
} else {
print("Image uploaded successfully to Dropbox")
// You can obtain the Dropbox URL for the uploaded image from the response metadata
if let metadata = response {
let dropboxUrl = metadata.pathDisplay
print("Dropbox URL: \(dropboxUrl)")
// Get shared link for the uploaded image from Dropbox
client.sharing.createSharedLinkWithSettings(path: dropboxUrl).response { response, error in
if let error = error {
print("Error retrieving shared link from Dropbox: \(error)")
} else if let result = response?.url {
let dropboxSharedLink = result
print("Dropbox shared link: \(dropboxSharedLink)")
}
}
}
}
} else {
print("Error: Dropbox client or file data is nil")
}
}
CDN transformation
Once the image is uploaded to cloud storage, you can generate CDN-optimized URLs through the image CDN platform. For example, you can use ImageKit to generate a CDN URL using the shared link of the image you generated when you stored your images in your cloud storage.
You can also use a service like Filestack to generate the CDN URL directly. For instance, you can use Filestack iOS SDK to integrate Filestack File Uploader in your app and upload images directly to your desired cloud storage platform, such as Dropbox. Once the file is uploaded successfully, Filestack returns a CDN URL that you can use to deliver your files:
https://cdn.filestackcontent.com/HANDLE
If the CDN service supports image optimization and transformation, you can also first transform the image before delivering it on your app. For example, here is how you can use the CDN URL returned by Filestack to apply transformations (smart crop in the below example):
https://cdn.filestackcontent.com/smart_crop=width:400,height:400/HANDLE
Updating iOS app logic
Finally, you have to modify your app logic to ensure that images load efficiently from the CDN instead of directly from your server or local storage. You can use a popular Swift networking library like Alamofire for this purpose. For instance, you can leverage AlamofireImage to efficiently load the image from the CDN-optimized URL and display it in an image view.
import AlamofireImage
// Load image from CDN URL using AlamofireImage
let imageUrl = "CDN_OPTIMIZED_URL"
Alamofire.request(imageUrl).responseImage { response in
if let image = response.result.value {
// Use the image in your app
imageView.image = image
}
}
Best practices for image delivery with free CDNs
- Implement lazy loading techniques to defer the loading of images until the user accesses or requests them.
- Display placeholder images or loading spinners for images to provide visual feedback to users while images are being loaded asynchronously.
- Implement client-side image caching mechanisms to optimize the loading of previously displayed images further. You can use a framework like NSCache, which provides in-memory caching solutions for efficiently storing and retrieving images.
- Tracking your free-tier bandwidth provided by free images CDN service to avoid unexpected costs. If you want more bandwidth, you can upgrade to a premium plan.
Filestack: A valuable partner for your iOS apps
Filestack is a comprehensive file management solution that offers robust tools and APIs for online file upload, transformation, and delivery. Here’s how you can leverage Filestack for your iOS apps:
Convenient integration and simplified uploads
Filestack offers a specialized iOS SDK that seamlessly integrates Filestack’s powerful file management services into your iOS mobile apps. The SDK is updated for iOS 16, allowing you to create powerful apps with Filestack for devices using iOS 16.
Using the SDK, you can integrate the Filestack File Uploader called the File Picker in your iOS app. The File Picker ensures secure, fast, and reliable file uploads. It supports multiple ways to upload files, including user-friendly drag-and-drop. The File Picker allows you to upload images from 20+ integrated sources, including Facebook, Instagram, Google Drive, AWS, and Dropbox. Moreover, you can integrate the uploader with your desired cloud storage and store your images there directly.
The File Picker currently supports the following cloud storage solutions:
- Google Cloud Storage
- Amazon S3
- Microsoft Azure
- Dropbox
- Rackspace
Filestack CDN
Once you upload an image through Filestack File Picker/Uploader, Filestack instantly returns a CDN URL. You can use this CDN URL to deliver images to your users quickly. Filestack CDN supports responsive images. Thus, it provides a consistent experience on any device. With Filestack CDN, you can deliver optimized images to iOS users worldwide regardless of their location.
Robust transformations
Filestack also offers advanced image optimization and transformation features through its Processing API. These include:
- Image compression
- Image resize
- Crop and smart crop
- Rotate, flip, and flop
- File type/format conversion
- Various image filters and enhancements
- Watermarks, and more.
You can apply these transformations using the CDN URL. For example, here is how you can automatically enhance an image:
https://cdn.filestackcontent.com/enhance/HANDLE
Conclusion
Images are an integral part of today’s modern mobile apps. However, mobile devices have limited storage capacity and storing images locally can slow down users’ devices and negatively affect the user experience. This is where cloud storage comes in. Cloud storage services offer virtually unlimited storage capacity, allowing you to store as many images as you want without worrying about storage capacity. Merging cloud storage solutions with free image CDNs can significantly boost your app’s performance and enhance the user experience. An image CDN stores copies of your app images on its various servers, which are distributed worldwide. Then, when a user requests the image, the CDN service delivers it from the server nearest to the user. This reduces latency and improves loading times.
Sign up for Filestack free and deliver images faster to users worldwide with its robust CDN!
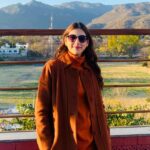
Sidra is an experienced technical writer with a solid understanding of web development, APIs, AI, IoT, and related technologies. She is always eager to learn new skills and technologies.
Read More →