As a web developer, you know file delivery and uploads are essential features of various modern web apps and websites. For instance, you would need a file uploader to upload different types of images to your website/app. Moreover, you often have to provide a file upload feature to end users, enabling them to upload images, documents, and videos to your app. While file uploading is a simple process for end users, handling and delivering these files seamlessly isn’t as simple for developers.
First off, you need to store the uploaded files somewhere before delivering them on your app. But, storing so many files on your web server can cause storage issues, slowing down your website. Then, you also have to ensure the uploaded files are secure and protected. Another challenge is to employ robust mechanisms to handle various file formats and types (images, documents, videos) smoothly. It’s also crucial to handle large files efficiently, prevent bandwidth issues, and ensure fast file delivery. With all these challenges, file delivery and uploads often seem daunting.
Fortunately, a framework like Angular can make file upload and delivery processes a whole lot easier for developers. This article will provide a thorough understanding of how Angular streamlines file delivery and document upload processes in modern web apps.
Leveraging Angular for file delivery and uploads
Angular is a feature-rich frontend framework for building dynamic web apps. It is packed with various built-in tools and features for file uploading, handling, and delivery.
File upload strategies
Angular provides convenient and efficient built-in mechanisms for handling file uploads. You can use standard HTML forms or utilize Angular’s reactive forms for more advanced options.
You can use the <input type=”file”> element with HTML forms to accept user-uploaded files. Angular allows you to interact with these elements and access their values through event binding and property binding. This is a straightforward way to enable users to upload files and handle those files.
However, it’s best to leverage Angular Reactive forms if you’re looking for advanced file upload and handling functionalities. They provide direct access to the underlying object model of the form.
Reactive forms give you more flexibility and control over the upload process. They allow for asynchronous file uploads, validation, and error handling. Moreover, Reactive forms are highly reusable, and more scalable and testable.
Data Management
Managing file metadata and other related data efficiently is essential when dealing with file uploads. Angular allows developers to handle file metadata, such as filename, type, and size, seamlessly. The framework can interact with various backend services, including databases, through HTTP requests or other protocols.
You can store the metadata and other relevant information in your local storage or databases. For example, you can save file metadata with associated user details or content categories. This enables efficient file retrieval and management.
API Interactions
By leveraging RESTful API endpoints on the backend, developers can ensure secure and seamless file transfers and manage uploaded content effectively. You can implement these API endpoints using a backend language like Node.js or Python. Moreover, you can utilize Angular’s built-in HttpClient module to interact with these endpoints and make HTTP requests.
The HTTP Client supports various HTTP methods, such as GET, POST, PUT, and DELETE. This enables Angular apps to communicate securely with backend servers, ensuring secure file transfer and data management.
Moreover, you can implement authentication and authorization mechanisms to ensure only authorised users access files. For instance, you can utilize secure authentication protocols and techniques such as JWT (JSON Web Tokens) or OAuth. Angular apps can send authentication tokens through HTTP requests to authenticate users with backend servers.
Implementing robust error handling and validation mechanisms on the server side is also imperative. This will help prevent malicious file uploads and ensure data integrity.
Optimized file delivery
Optimized file delivery significantly enhances the performance, user experience, and overall success of an app or website. For instance, it helps improve page load speed, enhances bandwidth efficiency, and reduces server load. Here are various ways to optimize file delivery:
File formats
When dealing with file uploads, choosing appropriate file formats according to a specific use case is crucial. It streamlines the uploading process, enhancing the user experience. For example, when handling image files, it’s best to use web-friendly formats like WebP, JPEG, and PNG. These formats offer good image quality with smaller file sizes, facilitating quick uploads. Similarly, it’s recommended to choose compressed formats like PDF or ZIP for documents and archives. These formats reduce file size and improve uploading speeds. In your Angular app, you can dynamically render different file formats based on application requirements.
However, users can upload files in various formats. Thus, it’s essential to convert files to your desired format before uploading them to your server or cloud storage. You can leverage Angular libraries and plugins for file conversions or optimizations as needed.
Progressive loading
Another way to enhance the file delivery and upload process and user experience is to implement progressive loading techniques. For instance, you can display previews for images. Moreover, you can initially display partial content while other aspects of the file load in the background.
Techniques for progressive loading include:
- Lazy loading: With lazy loading, you can initially load only the visible portion of the image while the rest is loaded as the user scrolls. Angular offers built-in support for lazy loading of components and content. Thus making it easier to implement progressive loading for images and other media files.
- Observables and async pipe: You can utilize Angular’s observables and async pipe to handle asynchronous data loading efficiently and provide real-time feedback to the user about the file upload status. This ensures a smooth and responsive user experience while files are being loaded progressively.
Content delivery networks (CDNs)
A CDN is essentially a network of globally distributed servers and data centres. In other words, a CDN is a network of numerous servers distributed worldwide. Various CDN services are available that you can use for your Angular app.
When you use a CDN for your app, it caches the app’s files and stores their copies in various servers. Then, when a user requests the file on your app, the CDN delivers it from the server nearest to the user.
Here are the key advantages of using a CDN in your Angular app:
- CDN significantly improves the file delivery speed by reducing latency and delivering files from the server nearest to the user.
- CDNs provide global reach with their massive network of servers located worldwide. Thus, you can deliver files to any user from any part of the world quickly.
- CDN allows you to reduce the load on your web server by offloading file delivery to the CDN’s servers.
- CDNs significantly enhance the user experience by ensuring quick and seamless file delivery.
Handling large files
Sometimes, users can upload large or big files. Efficient handling of such files is crucial to ensure a seamless file upload and delivery process.
Common issues with large files include:
- Large files require more storage
- They can slow down your server
- They require more time to upload
- When you transfer large files to the server or cloud storage in a single batch, it can take lots of time or result in failed uploads
- Large files can cause your server to malfunction, posing security threats
Here are some effective ways to handle large file uploads:
- Implement chucked file uploads
- Enable parallel file uploads
- Compress large files
- Store files in the cloud instead of the web server
- Implement mechanisms for asynchronous uploading. For instance, you can utilize reactive programming patterns to handle asynchronous file operations in your Angular apps.
Filestack: Simplifying and Boosting File Handling
If you’re looking for pre-built file upload and delivery solutions for your Angular app, you can leverage a service like Filestack. Filestack is a cloud-based service offering robust APIs and tools for file uploading, transformation, and delivery for web apps.
Here is how Filestack can simplify the file upload, handling, and delivery process:
Seamless Integration
Filestack offers an Angular SDK that enables developers to integrate Filestack service/functionality into their Angular apps seamlessly. “filestack-angular” is essentially a wrapper on filestack-js SDK, which allows you to integrate Filestack service using just a few lines of code. Almost everything you can do with filestack-js, you can also do using the Angular component.
Powerful uploads
Filestack provides a secure, fast, and reliable file uploader called File Picker. The File Picker supports various ways to upload files, including user-friendly drag-and-drop. It comes with a beautiful interface that can also be customized to match your app’s design requirements.
Filestack File Picker supports various file types. These include several image formats, document types, videos, and audio files. Moreover, the uploader allows users to upload images from 20+ integrated sources, including local device, Facebook, Instagram, Google Drive, AWS, Dropbox, and more.
Another good thing about Filestack is that it uploads and stores your files directly to cloud storage (S3 bucket). It also allows you to integrate your own cloud storage solution with the uploader and store your files there directly. Therefore, Filestack enables direct file uploads from the user’s device to your chosen cloud storage provider.
The File Picker currently supports the following cloud storage solutions:
- Amazon S3
- Google Cloud Storage
- Dropbox
- Microsoft Azure
- Rackspace
Transformations on demand
Filestack provides efficient ways to manipulate and transform files on the fly. You can use the Filestack Processing API to apply various enhancements and transformations. The Processing API is easy to use and supports a wide range of transformations, such as:
- Cropping
- Resizing
- File compression
- File type/format conversion
- Various image filters and enhancements
Speedy file delivery
Filestack leverages a reliable CDN to minimize latency and deliver files quickly to users worldwide. Once a user uploads a file through Filestack File Uploader/Picker, Filestack instantly returns a CDN URL. You can use this URL to deliver the file to users directly. You can also first transform the files through the Processing API and then deliver them to users.
Efficient handling of large file uploads
Filestack supports various techniques and mechanisms to handle large files efficiently. It supports parallel, chunked and asynchronous uploads. It also allows you to convert file formats or compress files before uploading. Additionally, Filestack has a powerful Content Ingestion Network (CIN), which significantly increases international upload speeds.
Code Examples (JavaScript/TypeScript)
Filestack File Upload
Here is a basic example of using Filestack File Uploader in your Angular app:
Once you’ve created an Angular project, create a component for filestack file upload functionality. We’ll name it Filestackcomponent.
Add the following code in your filestack.component.ts file:
import { Component, OnInit } from '@angular/core';
import { FilestackService } from '../service/filestack.service';
@Component({
selector: 'app-file-stack',
templateUrl: './file-stack.component.html',
styleUrls: ['./file-stack.component.css']
})
export class FileStackComponent implements OnInit {
constructor(private filestackService: FilestackService) {
}
message = ''
ngOnInit(): void {
}
upload() {
this.filestackService.client.picker().open()
}
}
Now, add the following code to your filestack.component.html file:
<form>
<div class="mb-3">
<label for="formFile" class="form-label">Upload with Filesteck example</label>
</div>
<button type="button" class="btn btn-primary" (click)="upload()">upload</button>
</form>
Next, create another component, name it ‘service’ and add the following code to the ts file. We’ll initialize our Filestack API here (Replace YOUR API KEY with your actual API key)
import { Injectable } from '@angular/core';
import {Client,init} from 'filestack-js';
@Injectable({
providedIn: 'root'
})
export class FilestackService {
client:Client
constructor() {
this.client = init('YOUR API KEY');
}
}
Now, add the following code to your app.module.ts file:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { FileStackComponent } from './file-stack/file-stack.component';
import { FormsModule } from '@angular/forms';
import { SimpleUploadComponent } from './simple-upload/simple-upload.component'; // Import FormsModule here
@NgModule({
declarations: [
AppComponent,
FileStackComponent,
],
imports: [
BrowserModule,
AppRoutingModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
You can try the above Angular app in Visual Studio Code. Once you open the app in your browser, it’ll show the following homepage:
When you click the upload button, it’ll open the Filestack File Uploader:
You can then choose and upload images:
Once a file is uploaded through Filestack File Uploader, Filestack returns a CDN URL for it. You can use the CDN URL to deliver files.
Here is the base URL for all assets on the Filestack CDN:
https://cdn.filestackcontent.com/HANDLE
Conclusion
Angular is a powerful framework for web development, allowing developers to build dynamic user interfaces. It also offers various powerful tools and mechanisms for streamlined file uploading, handling, and delivery. For instance, Angular supports built-in Reactive forms that provide advanced options for handling files. Angular also supports lazy loadings, observables, and async pipe, facilitating progressive loading. Utilizing a reliable third-party solution like Filestack can enhance the file delivery and uploading process further. Filestack complements Angular by minimizing backend overhead. Moreover, it adds robust file transformations and maximises delivery speed, particularly in high-volume cases.
Sign up for Filestack free and improve your file upload and delivery speed today!
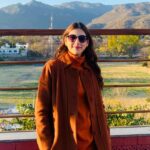
Sidra is an experienced technical writer with a solid understanding of web development, APIs, AI, IoT, and related technologies. She is always eager to learn new skills and technologies.
Read More →