Looking to make your web app handle files better through efficient file delivery? You’re in the right place! This blog will show you how to use JavaScript with Angular to process file delivery faster and more efficiently, all thanks to Filestack.
File delivery handling is important for web apps, especially when dealing with big files or complicated tasks. JavaScript and Angular can help you build apps that manage files really well. But putting them together and making everything work smoothly can be tough.
In this blog, we’ll start with the basics of JavaScript and how it fits with Angular for file tasks. Then, we’ll look at real examples of this combo’s awesomeness, like:
- Handling files smoothly when you transfer files on file request
- Creating custom tools for files
- Keeping everything secure to send files.
But wait, there’s more! We’ll also teach you how to make your file delivery process super easy with Filestack for large files. We’ll cover how to set it up, cool things you can do with it, and why using a global delivery network is a big win. Let’s begin.
How do you use Angular and JavaScript for high-performance file processing?
You can use Angular and JavaScript to ensure your web app handles files well. Here’s how:
JavaScript is a coding language that helps websites display pop-up messages or process files with password protection. It’s great for handling specific files because it can perform tasks without freezing or stopping the website.
The angular framework helps organize your code and make your web app run smoothly even with more storage and maximum size. It works well with JavaScript, especially with TypeScript. It is a type of JavaScript that helps make your code easier to understand and manage.
👉You can use Angular and JavaScript to manage files in a document system. Angular handles how things look on the screen, while JavaScript handles uploading, downloading, and converting files in the background. In a data visualization app, JavaScript can process large sets of data from files and show them coolly on the screen for users to interact with.
How do you optimize file operations with JavaScript and Angular?
To make file operations faster and safer in your web app browsers support, you can use JavaScript and Angular in smart ways:
Efficient file handling
JavaScript can handle files in device folder without slowing down your app. It can upload or download files in the background while your app runs smoothly.
// Example of asynchronous file upload in JavaScript
function uploadFile(file) {
return new Promise((resolve, reject) => {
const xhr = new XMLHttpRequest();
xhr.open('POST', '/upload', true);
xhr.onload = () => {
if (xhr.status === 200) {
resolve(xhr.responseText);
} else {
reject(xhr.statusText);
}
};
xhr.onerror = () => reject(xhr.statusText);
xhr.send(file);
});
}
Custom file processing modules
You can create special tools in your app for your file needs. For example, you could make a tool that changes file formats or checks if a file is okay.
// Example of a custom Angular service for file processing
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class FileProcessorService {
constructor() { }
processFile(file: File): Promise<string> {
// Custom file processing logic here
return new Promise<string>((resolve, reject) => {
// For demonstration, let's say we're converting the file to base64
const reader = new FileReader();
reader.onload = () => resolve(reader.result as string);
reader.onerror = (error) => reject(error);
reader.readAsDataURL(file);
});
}
}
Enhanced security: JavaScript and Angular have built-in features to keep your files and app safe. They can protect against things like hackers trying to steal your files or mess with your app.
// Example of Angular component with enhanced security for file handling
import { Component, SecurityContext } from '@angular/core';
import { DomSanitizer, SafeUrl } from '@angular/platform-browser';
@Component({
selector: 'app-file-handler',
templateUrl: './file-handler.component.html',
styleUrls: ['./file-handler.component.css']
})
export class FileHandlerComponent {
constructor(private sanitizer: DomSanitizer) { }
// Sanitizing file URLs for secure file handling
sanitizeFileUrl(fileUrl: string): SafeUrl {
return this.sanitizer.sanitize(SecurityContext.URL, fileUrl);
}
}
How do you streamline the complex file delivery workflow using Filestack?
Filestack allows integration with different programming languages and frameworks. When you visit the Filestack website, you will see a tab called “SDKs.” It is a dropdown that allows you to integrate with different technologies. An example is shown below:
Integration with Angular and JavaScript
When initiating Filestack with Angular, you may install it using NPM as under:
npm install filestack-js
npm install @filestack/angular
The next step is to include FilestackModule in your app.module.ts:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
import { FilestackModule } from '@filestack/angular';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
FilestackModule.forRoot({ apikey: YOUR_APIKEY, options: ClientConfig })
],
bootstrap: [AppComponent]
})
export class AppModule {}
Implement the API key inside your HTML file as follows:
<ng-picker-overlay
apikey="YOUR_API_KEY">
</ng-picker-overlay>
You can also access the Filestack file delivery module through our CDN under:
https://static.filestackapi.com/filestack-angular/{MODULE_VERSION}/filestack-angular.umd.min.js
Here is how you can map the file delivery to the module:
https://static.filestackapi.com/filestack-angular/{MODULE_VERSION}/filestack-angular.umd.min.js.map
You may check examples with more details at https://github.com/filestack/filestack-angular
How does Filestack’s API complement JavaScript for efficient file handling?
Filestack’s API works well with JavaScript and Angular to handle files efficiently. Here’s how:
- It helps you easily upload files from different sources like your computer or the internet.
- You can store uploaded files securely in the cloud or on your own servers.
- It lets you transform files, like resizing images or converting formats, to suit your needs.
- If you’re using Angular, Filestack provides tools to integrate their API into your app more easily.
- You can use Filestack’s API directly with simple code for JavaScript apps.
Advanced file manipulation
Filestack has special tools that help change and manage files. Here’s how JavaScript can make these tools work even better:
1. Transformations
Filestack can make images smaller or change their shape. JavaScript helps make these changes happen smoothly. You can use JavaScript to resize an image to fit better on a webpage.
const client = filestack.init('YOUR_API_KEY');
const result = client.transform('YOUR_IMAGE_URL', { resize: { width: 300, height: 200 } });
console.log(result.url);
2. Smart Compression
Filestack can make files smaller without losing quality. JavaScript helps make this compression process work well.
const client = filestack.init('YOUR_API_KEY');
const result = client.transform('YOUR_IMAGE_URL', { compress: true });
console.log(result.url);
3. File Conversions
Filestack can convert a file from one type to another, and JavaScript can control this conversion process.
const client = filestack.init('YOUR_API_KEY');
const result = client.convert('YOUR_FILE_URL', 'pdf');
console.log(result.url);
4. Customization
Filestack lets you customize how files are changed. JavaScript can help you personalize these changes.
const client = filestack.init('YOUR_API_KEY');
const result = client.transform('YOUR_IMAGE_URL', { filters: { sepia: true } });
console.log(result.url);
Global delivery network
Filestack’s CDN (Content Delivery Network) helps quickly and reliably distribute processed files worldwide:
👉Files are stored on servers closer to users for quicker access.
👉It ensures files are always available, even during high traffic or server issues.
👉Servers are located worldwide for fast file delivery to users everywhere.
👉It uses smart techniques to speed up file loading and save bandwidth.
👉Files are delivered securely with encryption and access controls.
Code Examples
Angular component for file processing
You can follow these steps to create an Angular component that processes files using JavaScript and then uses Filestack for storage and delivery.
First, ensure you have the Filestack SDK installed in your Angular project:
npm install filestack-js
Then, you can create a component like the one below:
file-processing.component.html:
<input type="file" (change)="onFileSelected($event)">
<button (click)="uploadFile()">Upload</button>
file-processing.component.ts:
import { Component } from '@angular/core';
import * as filestack from 'filestack-js';
@Component({
selector: 'app-file-processing',
templateUrl: './file-processing.component.html',
styleUrls: ['./file-processing.component.css']
})
export class FileProcessingComponent {
fileToUpload: File | null = null;
onFileSelected(event: any): void {
this.fileToUpload = event.target.files[0];
}
uploadFile(): void {
if (!this.fileToUpload) {
console.error('No file selected.');
return;
}
// Process the file using JavaScript (e.g., resize an image)
// For demonstration purposes, we'll just upload the file to Filestack
const client = filestack.init('YOUR_API_KEY');
client.upload(this.fileToUpload)
.then((response) => {
console.log('File uploaded:', response);
alert('File uploaded successfully!');
})
.catch((error) => {
console.error('File upload error:', error);
alert('Error uploading file. Please try again.');
});
}
}
In this component, the `onFileSelected` method is called when a file is selected using the file input element. The `uploadFile` method processes the selected file (in this case, simply uploads it to Filestack). Replace `’YOUR_API_KEY’` with your actual Filestack API key.
JavaScript module example
You can follow this example to create a simple JavaScript module for basic file edits like image cropping before upload. This module will use the Filestack API to upload and crop images.
First, ensure you have the Filestack SDK included in your project. You can use a CDN link in your HTML file:
<script src="https://static.filestackapi.com/filestack-js/3.x.x/filestack.min.js"></script>
Then, create a JavaScript module:
// fileEditModule.js
const fileEditModule = (function () {
let filestackApiKey = 'YOUR_API_KEY';
let client = filestack.init(filestackApiKey);
function cropImage(file, cropOptions, successCallback, errorCallback) {
const options = {
crop: cropOptions,
mimetype: 'image/jpeg', // Adjust mimetype as needed
maxSize: 1024 1024 5, // 5MB limit, adjust as needed
};
client.upload(file, options)
.then(response => {
successCallback(response);
})
.catch(error => {
errorCallback(error);
});
}
return {
cropImage: cropImage
};
})();
In this module, the `cropImage` function takes:
- File object
- Crop options (e.g., coordinates and dimensions)
- Success callback
- Error callback.
It uploads the file to Filestack with the specified crop options.
You can then use this module in your HTML file like this:
<input type="file" id="fileInput">
<button id="cropButton">Crop and Upload</button>
<script src="fileEditModule.js"></script>
<script>
document.getElementById('cropButton').addEventListener('click', function () {
const fileInput = document.getElementById('fileInput');
const file = fileInput.files[0];
// Example crop options (adjust as needed)
const cropOptions = {
x: 100,
y: 100,
width: 200,
height: 200
};
fileEditModule.cropImage(file, cropOptions,
function (response) {
console.log('File uploaded:', response);
alert('File uploaded successfully!');
},
function (error) {
console.error('File upload error:', error);
alert('Error uploading file. Please try again.');
});
});
</script>
👉Replace `’YOUR_API_KEY’` with your actual Filestack API key.
File Delivery: Conclusion
Boosting your web app’s performance means making it faster and more efficient for file delivery. Integrating JavaScript with Angular allows for advanced file delivery, and when you add Filestack to the mix, you get a powerful combination for handling files.
JavaScript is a programming language that runs in the browser and can be used to create interactive elements on web pages. Angular is a popular framework for building web applications that provides a structured way to manage your code.
Filestack is a service that provides tools for uploading, transforming, and delivering files. By integrating Filestack with Angular and JavaScript, you can build web apps that easily handle files.
File Delivery: FAQs
How to send 50 GB files online for free?
You can use free services like Filestack to send 50GB files for free online.
What is meant by file sharing?
File-sharing services help us share files online, such as social media, emails, or cloud services.
How do I send a 10 GB file?
Use Filestack to send a 10 GB file.
Is it reliable to trust Filestack for efficient file delivery?
Filestack has reliable security protocols to help you get a trustworthy file delivery experience.
Sign up for free at Filestack today for efficient file delivery.
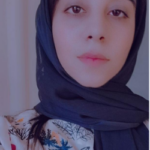
Ayesha Zahra is a Geo Informatics Engineer with hands-on experience in web development (both frontend & backend). Also, she is a technical writer, a passionate programmer, and a video editor. She is always looking for opportunities to excel in her skills & build a strong career.
Read More →