Efficient file handling has become developers’ main focus in developing React applications. There are many of the latest trends and features one needs to have in his React application. Today, we will discuss streamlined file downloads using React download file from API. We will cover the basics of file downloading in React. Besides, we will also explore the React downloading challenges and React API file handling.
We will also show you the implementation of an API to enable React downloads. At the same time, you will also learn about the benefits of using React API file handling. Finally, we will share some best practices to optimize file downloading in React. Let’s begin.
What are the basics of file downloading in React?
In React, file downloading involves retrieving and saving files from a server to the user’s device. This fundamental process is integral for applications dealing with documents, images, or any downloadable content.
Consider it as a digital transfer where React communicates with a server to fetch a file. Then, it seamlessly presents the option to save or open it.
Challenges often revolve around optimizing download speeds and efficiently managing large or multiple files. Let’s explore the challenges linked with file downloading in React.
What are the common challenges of file downloading in React?
File downloading in React comes with its share of challenges. Those challenges are listed below:
One common issue is the potential for slow download speeds. Slow speeds are always impacting user experience.
Handling bulk or large files can also affect performance.
Ensuring seamless asynchronous operations poses another challenge. This is because the file downloads can potentially block the main thread. As a result, it can lead to unresponsive interfaces.
Robust error handling is also important to manage network issues or server errors during the download process.
Security considerations have become essential to ensure the safety of sensitive information. Some examples are encrypting data transmission and implementing authentication checks.
We must address the above challenges to ensure fast file uploading in our React application.
How can APIs be utilized for downloading files in React?
Using APIs for file downloads introduces an efficient approach to handling data transfer between servers and clients. APIs help us simplify the process of fetching and downloading files. Technically, developers can utilize HTTP clients like Axios to interact with APIs and retrieve file data as blobs. This allows for a structured and organized exchange of information.
As a result, APIs are highly useful for file downloading in React applications. One such example is Filestack.
Let’s explore some amazing benefits of using APIs for downloading files in React.
What are the benefits of using APIs for downloading files in React?
- Firstly, APIs offer a standardized method of communication. As a result, it ensures seamless integration and interoperability.
- APIs provide a customizable and optimized solution. It enables developers to match file handling to the specific application needs.
- Additionally, APIs enhance scalability and maintainability. This makes it easier to adapt to evolving project requirements. This approach fosters a more robust and responsive file download experience for React applications.
How to implement file downloads in React using APIs
Here are the steps to implement file downloads in React using APIs. Let’s begin.
1. Install Filestack
Firstly, install the Filestack library by running the following command in your React project:
npm install filestack-js
2. Initialize Filestack
In your React component, import and initialize Filestack with your API key:
import filestack from 'filestack-js';
const apiKey = 'ADD_YOUR_FILESTACK_API_KEY';
const client = filestack.init(apiKey);
Please note that you must register yourself at Filestack to get the API key.
Create a File Download Function
Define a function to initiate the file download using Filestack. This example assumes you have a button triggering the download:
const downloadFile = async () => {
try {
const fileUrl = 'URL_TO_YOUR_FILE_ON_FILESTACK';
const { url } = await client.retrieve(fileUrl);
// Use the file URL as needed (e.g., open in a new tab or trigger a download)
window.open(url, '_blank');
} catch (error) {
console.error('Error downloading file:', error);
}
};
Implement the Download Button
Create a React component with a button to trigger the file download:
import React from 'react';
const DownloadButton = () => {
return (
<button onClick={downloadFile}>Download File</button>
);
};
export default DownloadButton;
Render the Component
Integrate the `DownloadButton` component within your application:
import React from 'react';
import DownloadButton from './DownloadButton';
const App = () => {
return (
<div>
<h1>Your React App</h1>
<DownloadButton />
</div>
);
};
export default App;
How to optimize React download file from API for better performance and user experience?
Optimizing performance in React download file from API is an important step. It helps us achieve an excellent user experience.
Firstly, you should use asynchronous operations. These operations help prevent blocking the main thread. As a result, it ensures responsiveness during downloads.
Implementing robust error handling is also important. For this purpose, you must provide clear feedback to users in case of network issues or server errors.
Additionally, prioritize security by encrypting data transmission and implementing proper authentication checks. These practices can help us safeguard sensitive information.
What are the case studies where efficient file downloads have significantly impacted?
There are many case studies around us for React download file from API. Let’s take a look at how Filestack helped some companies to enhance file downloads using React download file from API.
The Imagine Learning Classroom lesson player is a helpful tool for both teachers and students in grades K-12. It helps them learn different subjects like math, English, science, and history. When teachers create lessons using IL Classroom, they can use Filestack to add things like slides or cards. This makes it easy to upload files from their computer or Google Drive.
The cool thing is that teachers can also record themselves talking about the lesson. This practice is useful for online teaching. The Imagine Learning Classroom is made to support teachers and make it easier for them to teach. It is more helpful, especially during times like the pandemic when they had to teach remotely.
React download file from API: Conclusion
In the above article, we discussed the importance of file downloads in React. At the same time, we also talked about implementing file downloading in React using the API. We showcased the easy integration of a stack with React applications to implement file downloads. In the end, we shared some best practices to achieve better file downloading. Moreover, we also presented a case study of a popular platform for effective file downloading.
FAQs
What makes APIs essential for file downloading in React applications?
APIs help handle the transfer of information between servers and users’ devices. They make it easy to get, download, and organize files. APIs also give you ways to customize and improve how the process works. So, they’re helpful tools that simplify the whole file-handling thing in React. For a refresher course on uploading take a look at our html file upload blog.
How do APIs enhance the performance of file downloads in React?
APIs can improve how things work by letting files download asynchronously. It means they can happen at the same time without slowing things down. This helps lighten the load on the server. It also gives you more control over how files are handled. The cool part is that this leads to faster download speeds.
Are there any security considerations when using React download file from API?
We must implement data encryption and user authorization techniques to ensure secure API file downloads in React Download file from API.
How do you integrate download functionality in React code?
In React Download file from API, integrate file download through libraries like Axios. Moreover, you should also incorporate a button-triggered function for a seamless user experience.
Sign Up for free at Filestack today – Handle React downloads with efficiency.
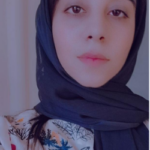
Ayesha Zahra is a Geo Informatics Engineer with hands-on experience in web development (both frontend & backend). Also, she is a technical writer, a passionate programmer, and a video editor. She is always looking for opportunities to excel in her skills & build a strong career.
Read More →