File downloads have become integral to many web applications in today’s digital world. Developers often rely on APIs to download images, documents, or multimedia files. As a result, it can help them facilitate smooth and efficient file retrieval. Regarding React applications, one powerful tool for React download file is the Filestack API. Hence, this is the best option for React download file from API requests.
In this blog post, we will explore the concept of downloading files from the Filestack API in React. Moreover, we will also explore the significance it holds in modern web development & React download file, at the same time. We will delve into the challenges of implementing file downloads through an API in a React application and discuss potential solutions.
Downloading files from the Filestack API in React offers several advantages. Moreover, it provides a streamlined approach to handling complex file operations. Hence, it ensures the efficient delivery of files to users.
However, implementing file downloads from an API in a React application can present certain challenges. These challenges may include managing authentication tokens, handling file size limitations, and ensuring compliance with cross-origin resource sharing (CORS). Moreover, it also includes dealing with various file formats. Let’s learn more about these concepts below.
What is the Filestack file uploader?
Filestack’s file uploader also comes with a file uploading API. Moreover, this powerful uploader lets you upload, transform, and deliver any file. At the same time, you can integrate Filestack’s powerful APIs into your React applications. This API gives you a beautiful user interface with over 20 integrated sources. Moreover, the content ingestion network in Filestack makes it 100 times more reliable.
At the same time, you can ensure the safety of users through the secure transfer provided by Filestack. Filestack’s file uploader also comes with SDKs. These SDKs are helpful for the quick integration of your React apps.
Apart from the above features, it would be best not to forget the URL-based file transformations. Moreover, it allows you to transform your file by changing only a few parameters inside a URL. One example is given below.
https://cdn.filestackcontent.com/key-here/resize=height:200/file-id-here
There is much more to explore at Filestack.
Visit Filestack now to check out all the amazing features.
How to use Filestack to streamline file downloads in React?
Since we are talking about the integration of Filestack with React app, let’s check how it works. Filestack ensures the simplest file downloads in React. Here are the reasons:
It allows drag and drop to simplify the file-handling process.
You get options to store and keep your files in cloud storage. Some options supported by Filestack are:
- Amazon S3
- Rackspace
- Dropbox
- Azure Blob Storage
- Google Cloud Storage
Filestack allows you to remove watermarks from the images. Moreover, you can also resize and crop them just after uploading them at Filestack.
The processing engine at Filestack is a powerful system that helps you handle any file.
You can transform the images with a beautiful user interface with Transformations UI.
Let’s check out the Filestack UI component.
What is the Filestack UI component?
As mentioned, Filestack’s transformations UI component helps us get a modern UI. Moreover, it allows the users to adjust image sizes, apply filters, and perform other transformations. Hence, you get a seamless user experience.
To integrate it into your React application, use the following UMD module const link.
<script src="https://static.filestackapi.com/transforms-ui/2.x.x/transforms.umd.min.js"></script>
<link rel="stylesheet" href="https://static.filestackapi.com/transforms-ui/2.x.x/transforms.css" />
You must create a container to display image transformation results for file name.
<div classname="text-align:center;">
<img id="result" style="width:600px" />
</div>
Then, you must initialize the transformation UI & create URL as under.
const tr = new FilestackTransform('API_KEY')
tr.open(FILE_URL).then(res => { // replace FILE_URL with the link to the image
document.getElementById('result').src = res // display result of the transformations
})
Here is the output.
How does Filestack file download management work?
Filestack offers comprehensive features and functionalities for effective file download management. Let’s explore some key aspects of how Filestack handles file downloads to enhance the user experience and optimize file retrieval.
Generating secure download links
Filestack enables developers to generate secure download links for files. Moreover, these links contain access tokens and authentication mechanisms that ensure only authorized users can download the files.
Tracking download statistics and analytics
Filestack provides powerful analytics tools that allow developers to track and monitor file downloads.
React download file from API: Resumable downloads for handling large files
One of the challenges of downloading large files is the potential for interruptions or network failures. Moreover, Filestack addresses this by offering resumable downloads. Hence, it allows users to resume interrupted downloads from where they left off.
Can we integrate the Filestack API into a React app?
Integration with Filestack is very simple and easy. You have to create an account and get your API key. Once you get your API key, add it to the following code. Interestingly, only two lines of source code are enough to integrate Filestack into your app.
<script src="//static.filestackapi.com/filestack-js/3.x.x/filestack.min.js"></script>
const client = filestack.init(YOUR_API_KEY);
client.picker().open();
However, you can also use SDKs to integrate Filestack into your React application. Complete documentation is available on Filestack, which gives you a Filestack-React GitHub repository. Finally, you can easily send an API request for downloading files through the Filestack backend API.
React download file from API: Install the Filestack SDK
Install the Filestack SDK in your React app. Furthermore, you can use a package manager like npm or yarn.
React download file from API: Configure the SDK
This step typically involves importing the SDK and providing your API key as a parameter when initializing it. Moreover, this allows the SDK to authenticate your requests to the Filestack API.
React download file from API: Use the Filestack API functions
With the SDK configured, you can now use the Filestack API functions to perform various operations such as file uploads, transformations, and file downloads. Moreover, the API functions provide methods to generate secure download links, track download statistics, and handle resumable downloads.
What are the best practices and considerations for file downloads?
Security considerations when handling file downloads
- Use authentication tokens or secure download links to authenticate and authorize users.
- Validate file types, sizes, and formats before allowing them to be downloaded.
- Use secure protocols such as HTTPS to encrypt the file transfer between the server and the client.
Performance optimization techniques for efficient downloads
- Compress files before downloading to reduce their size and optimize transfer speed.
- Implement caching mechanisms to store frequently accessed files on the client or server side.
- Allow parallel downloads by utilizing techniques like multi-threading or chunked downloading.
- Utilize CDNs to distribute files across multiple servers worldwide.
Handling errors and fallback options for failed downloads
- Implement robust error-handling mechanisms to handle network failures, server errors, or file not found scenarios.
- Implement retry logic for failed downloads to automatically attempt downloading again after a certain period.
- Provide alternative download options such as alternative file formats, lower-quality versions, or alternative download sources.
React download file from API: Final thoughts
In this blog post, we have explored simplifying React file downloads through API-based file retrieval using the Filestack API. Moreover, we highlighted the importance of leveraging the Filestack API for efficient file management in React applications.
From generating secure download links to tracking download statistics and enabling resumable downloads for large files, Filestack offers a comprehensive solution for file download management.
By integrating the power of the Filestack API into React, developers can simplify file downloads, enhance security, and improve the overall performance of their applications.
React download file from API: FAQs
How do I download a local file in React?
To download a local file in React, use the HTML5 download attribute or utilize a library-like file-saver.
How do I get a file in React?
To get a file in React, you can use the File API or libraries like Filestack for simplified file handling.
How do I download a text file from React JS?
You can use the “download” attribute with an anchor tag to download a text file in React JS.
To create a download button in React, use an HTML anchor tag with a “download” attribute.
Unlock the Power of Filestack Today and Simplify Your File Management Workflow!
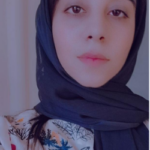
Ayesha Zahra is a Geo Informatics Engineer with hands-on experience in web development (both frontend & backend). Also, she is a technical writer, a passionate programmer, and a video editor. She is always looking for opportunities to excel in her skills & build a strong career.
Read More →