Every day, thousands of people worldwide download different types of files from the web, from images, videos, and audio files to plain text files. As a result, file downloads have become an integral part of many websites and web apps. However, adding file upload functionality to websites or web apps can be challenging for developers because they must provide a seamless user experience while ensuring security, speed, cross-browser compatibility, and more. Fortunately, developers can efficiently retrieve and download files in their web apps using an API. Since React is one of the most used JavaScript frameworks for web development, React download files from API is quite common.
This article will explore different aspects of React download files using an API. We’ll also discuss how Filestack can help you with efficient file download and retrieval in your React apps.
How do file downloads from the web work?
In general, the process of downloading files from the internet is as follows:
- The client, which is the user’s web browser, sends an HTTP request to the server to download a specific file.
- The server responds by returning the contents of the file to be downloaded, as well as instructional HTTP headers stating how the browser should download the file.
- The browser starts the file download, depending on the user’s settings. For example, browsers typically ask for the user’s permission before downloading a file.
Nearly all file downloads from the web make use of HTTP (Hypertext Transfer Protocol). HTTP is essentially a set of rules for transferring information or files over the web. HTTP can transfer various file types, including images, videos, text, and audio files. In other words, HTTP enables the transfer of files or information between the client/web browser and the web server.
Enabling file downloads in a website or web app
From the developers’ point of view, there are various approaches to enabling file downloads in a website or web app. For instance, you can download files via direct links or using API endpoints.
The problem with downloading a file via a URL/direct link is that we first have to generate the file on the server side, and once the file is ready, we can provide a URL to the user to download the file. This means we will have a file on the server that’ll take up space, which can cause performance issues.
The best option is to use an API to download files directly on the client side. With this method, we first get the file data via the API, then interpret it, and finally download it on the client side without the need to open a new tab. We can download various file types using an API, such as PDF, PNG, CSV, and ZIP.
React download file from API: How to fetch file from API?
When it comes to React download files from API, we have multiple options. We can use Axios for API calls or Fetch API. Axios is essentially a library in React used to send HTTP requests to REST API or API endpoints. With Fetch API, we can make HTTP requests to the server/backend using the fetch() method.
Assuming that you have set up your website’s backend and have created a React project as well as a file download button, you can use the following code for making HTTP request to the API (we’re using the Fetch API method):
handleSubmit = (event) => {
this.setState({
errors: null,
loading: true,
}, () => {
Helpers.httpRequest(
`http://localhost:5000?file=${this.state.file}`,
'get',
)
.then((response) => {
this.setState({
loading: false
});
})
.catch((error) => {
error.json().then((json) => {
this.setState({
errors: json,
loading: false
});
})
});
});
event.preventDefault(); //stops the defual action from occuring
}
Here, we’ve created a separate helpers file and kept out http requests there:
const Helpers = {
httpRequest: (url, method, payload, headers) => {
const conf = {
method,
headers: {
'Content-Type': 'application/json'
}
};
if (method.toLowerCase() === 'post' && payload && payload.length > 0) {
conf.body = JSON.stringify(payload);
}
return fetch(
url,
conf
).then((response) => {
if (response.ok) {
let contentdata = response;
if (response.headers.get('Content-Type').indexOf('application/json') > -1) {
contentdata = response.json();
}
return contentdata;
}
return Promise.reject(response);
});
},
};
export default Helpers;
React download file from API: How to handle file data?
We’ll now convert the file data into ‘blob’. Blob stands for “Binary Large Object” and holds the data stored in a file, such as an image, GIF, video, or audio. It basically holds binary data as a single entity.
.then((response) => response.blob())
.then((blob) => {
React download file from API: How to generate downloadable links?
The next step in React download file from API is generating a downloadable link. We’ll create a downloadable able link using blob and URL.createObjectURL(), which essentially creates a string consisting of a URL that represents the object present in the parameter.
const fileurl = window.URL.createObjectURL(new Blob([blob]));
const link = document.createElement('a');
link.href = fileurl;
link.setAttribute('download', `sample.${this.state.file}`);
Next, we’ll append to HTML page and enable force download:
document.body.appendChild(link);
link.click();
All modern web browsers, such as recent versions of Chrome, Firefox, Opera, Safari, and Edge, support blob URLs.
React download file from API: How to enhance the user experience?
Here are some of the ways to enhance the user experience during file downloads:
- Display loading indicators while the requested file is generated.
- Show download progress.
- Display download errors (if any) and show alert messages in case of failed downloads.
- If there are any download errors, handle the errors efficiently to ensure a good user experience.
- Enable users to save the download file with their preferred filename.
- Implement mechanisms to enable users to pause and resume file downloads.
What are the best practices for file downloading?
Error handling
Some potential error scenarios with file download include network failure, file not found, or server error. Regarding network failure, implement a mechanism for resumable downloads or enable users to retry.
For server errors and files not found, check your file download functionality to see if there are any errors in the code. Another way to handle file download errors is to provide alternative download options, such as reduced file size, alternate file formats, etc.
Moreover, testing your file download functionality is essential before making it available for end-users. You create various test cases to ensure all file download features work correctly. For example, you can test whether the download button is working, check whether the downloaded and original file sizes are the same, etc.
Performance optimization techniques
- Reduce file size by compressing it to optimize transfer speed.
- Enable parallel downloads by implementing techniques like chunked downloads or multi-threading.
- Use CDNs to save copies of files in various servers worldwide to deliver content to the user quickly from the server nearest to the user.
- If files are not meant for public download, create secure download links and authenticate and authorize users.
- Encrypt files using HTTPS.
- Validate file format, types, and sizes, before enabling users to download them.
What is Filestack, and how can it help you with React file download?
Filestack provides a wide range of tools and APIs that help you with file upload, transformation, and delivery. It offers several UI integrations to enable you to deliver exceptional experiences to your end users. Moreover, developers can utilize various Filestack APIs as per their needs.
React File Upload
With Filestack File Uploader or File Picker, you can implement the file upload functionality in your apps with just a few lines of code. The File Uploader has a beautiful drag-and-drop interface and supports over 20 integrated sources.
Moreover, you can configure the uploader for multiple file uploads and show a progress bar for each file.
Filestack also offers a React File Upload SDK that you can use to add file upload functionality to your React app quickly:
First, install the SDK through NPM:
npm install filestack-react
Next, insert into your app:
import { PickerOverlay } from 'filestack-react';
<PickerOverlay
apikey={YOUR_API_KEY}
onSuccess={(res) => console.log(res)}
onUploadDone={(res) => console.log(res)}
/>
Here is how you can render a basic Overlay Picker
<PickerOverlay apikey=’YOUR_APIKEY’/>
File storage
Filestack stores all the files uploaded through the File Picker in an internally managed S3 bucket by default. Filestack also enables users to integrate their existing cloud storage solution with the uploader and store their files there.
File download and delivery
Filestack uses a CDN (Content Delivery Network) to ensure fast and reliable file downloads, retrieval, and delivery. When you upload a file through Filestack File Uploader, it instantly returns a CDN URL that you can use to download, retrieve, and deliver files. You can even use the CDN URL to transform files, such as compress images with React.
Here is the base URL for all your assets:
https://cdn.filestackcontent.com/HANDLE
Filestack also offers a File API that you can use to store, download, retrieve, overwrite, and delete files. For file download and delivery, we recommend you use CDN as it’s more efficient and reliable.
React download file from API: Final thoughts
File download is an essential feature of many React apps and websites. This means developers must enable end users to download various file types, such as text files, images, videos, etc. One efficient way is to enable React file downloads using an API. With this method, we get the file data using the API, then interpret it and download it directly on the client side. We can download different file types using an API, such as PNG, PDF, CSV, and ZIP.
If you’re looking for an easy React file upload and download solution, try Filestack today!
Frequently asked questions (FAQs)
What is the best way to handle file downloads in React?
The most efficient way to handle file downloads in React is using an API. With this method, you can download files directly on the client side.
What security considerations should you consider when downloading files from an external API?
Regarding security, you should validate file size, format, and type. Moreover, you must implement authorization and authentication techniques.
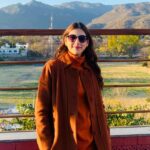
Sidra is an experienced technical writer with a solid understanding of web development, APIs, AI, IoT, and related technologies. She is always eager to learn new skills and technologies.
Read More →